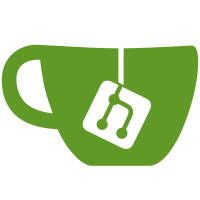
This allows users to add patches as attachments to merge request created via email. When an email to create a merge request is sent, all the attachments ending in `.patch` will be applied to the branch specified in the subject of the email. If the branch did not exist, it will be created from the HEAD of the repository. When the patches could not be applied, the error message will be replied to the user. The patches can have a maximum combined size of 2MB for now.
78 lines
2.4 KiB
Ruby
78 lines
2.4 KiB
Ruby
require "spec_helper"
|
|
|
|
describe EmailReceiverWorker, :mailer do
|
|
let(:raw_message) { fixture_file('emails/valid_reply.eml') }
|
|
|
|
context "when reply by email is enabled" do
|
|
before do
|
|
allow(Gitlab::IncomingEmail).to receive(:enabled?).and_return(true)
|
|
end
|
|
|
|
it "calls the email receiver" do
|
|
expect(Gitlab::Email::Receiver).to receive(:new).with(raw_message).and_call_original
|
|
expect_any_instance_of(Gitlab::Email::Receiver).to receive(:execute)
|
|
|
|
described_class.new.perform(raw_message)
|
|
end
|
|
|
|
context "when an error occurs" do
|
|
before do
|
|
allow_any_instance_of(Gitlab::Email::Receiver).to receive(:execute).and_raise(error)
|
|
end
|
|
|
|
context 'when the error is Gitlab::Email::EmptyEmailError' do
|
|
let(:error) { Gitlab::Email::EmptyEmailError }
|
|
|
|
it 'sends out a rejection email' do
|
|
perform_enqueued_jobs do
|
|
described_class.new.perform(raw_message)
|
|
end
|
|
|
|
email = ActionMailer::Base.deliveries.last
|
|
expect(email).not_to be_nil
|
|
expect(email.to).to eq(["jake@adventuretime.ooo"])
|
|
expect(email.subject).to include("Rejected")
|
|
end
|
|
end
|
|
|
|
context 'when the error is Gitlab::Email::AutoGeneratedEmailError' do
|
|
let(:error) { Gitlab::Email::AutoGeneratedEmailError }
|
|
|
|
it 'does not send out any rejection email' do
|
|
perform_enqueued_jobs do
|
|
described_class.new.perform(raw_message)
|
|
end
|
|
|
|
should_not_email_anyone
|
|
end
|
|
end
|
|
|
|
context 'when the error is Gitlab::Email::InvalidAttachment' do
|
|
let(:error) { Gitlab::Email::InvalidAttachment.new("Could not deal with that") }
|
|
|
|
it 'reports the error to the sender' do
|
|
perform_enqueued_jobs do
|
|
described_class.new.perform(raw_message)
|
|
end
|
|
|
|
email = ActionMailer::Base.deliveries.last
|
|
expect(email).not_to be_nil
|
|
expect(email.to).to eq(["jake@adventuretime.ooo"])
|
|
expect(email.body.parts.last.to_s).to include("Could not deal with that")
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
context "when reply by email is disabled" do
|
|
before do
|
|
allow(Gitlab::IncomingEmail).to receive(:enabled?).and_return(false)
|
|
end
|
|
|
|
it "doesn't call the email receiver" do
|
|
expect(Gitlab::Email::Receiver).not_to receive(:new)
|
|
|
|
described_class.new.perform(raw_message)
|
|
end
|
|
end
|
|
end
|