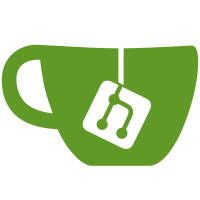
GitLab keeps a cache of the rendered HTML for a repository's README as stored in the HEAD branch. However, it was not used in all circumstances. In particular, the new blob viewer framework bypassed this cache entirely. This MR ensures a ::ReadmeBlob is returned instead of a ::Blob when asking a repository for an individual blob, if the commit and path match the readme for HEAD. This makes the cached HTML available to consumers, including the blob viewer. The ReadmeBlob is a simple delegator to the Blob, so should be compatible in all cases. Adding the rendered_markdown method is the only additional behaviour it contains.
67 lines
1.4 KiB
Ruby
67 lines
1.4 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
class Tree
|
|
include Gitlab::MarkupHelper
|
|
include Gitlab::Utils::StrongMemoize
|
|
|
|
attr_accessor :repository, :sha, :path, :entries
|
|
|
|
def initialize(repository, sha, path = '/', recursive: false)
|
|
path = '/' if path.blank?
|
|
|
|
@repository = repository
|
|
@sha = sha
|
|
@path = path
|
|
|
|
git_repo = @repository.raw_repository
|
|
@entries = Gitlab::Git::Tree.where(git_repo, @sha, @path, recursive)
|
|
end
|
|
|
|
def readme_path
|
|
strong_memoize(:readme_path) do
|
|
available_readmes = blobs.select do |blob|
|
|
Gitlab::FileDetector.type_of(blob.name) == :readme
|
|
end
|
|
|
|
previewable_readmes = available_readmes.select do |blob|
|
|
previewable?(blob.name)
|
|
end
|
|
|
|
plain_readmes = available_readmes.select do |blob|
|
|
plain?(blob.name)
|
|
end
|
|
|
|
# Prioritize previewable over plain readmes
|
|
entry = previewable_readmes.first || plain_readmes.first
|
|
next nil unless entry
|
|
|
|
if path == '/'
|
|
entry.name
|
|
else
|
|
File.join(path, entry.name)
|
|
end
|
|
end
|
|
end
|
|
|
|
def readme
|
|
strong_memoize(:readme) do
|
|
repository.blob_at(sha, readme_path) if readme_path
|
|
end
|
|
end
|
|
|
|
def trees
|
|
@entries.select(&:dir?)
|
|
end
|
|
|
|
def blobs
|
|
@entries.select(&:file?)
|
|
end
|
|
|
|
def submodules
|
|
@entries.select(&:submodule?)
|
|
end
|
|
|
|
def sorted_entries
|
|
trees + blobs + submodules
|
|
end
|
|
end
|