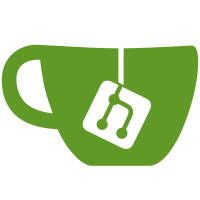
In 12.0, we turned the feature flag on that effectively turned off the --max-count flag for the count diverging commits call. Since we have commit graphs turned on, this did not affect preformance negatively. Thus, we want to deprecate the call that passes --max-count
32 lines
825 B
Ruby
32 lines
825 B
Ruby
# frozen_string_literal: true
|
|
|
|
module Branches
|
|
class DivergingCommitCountsService
|
|
def initialize(repository)
|
|
@repository = repository
|
|
@cache = Gitlab::RepositoryCache.new(repository)
|
|
end
|
|
|
|
def call(branch)
|
|
diverging_commit_counts(branch)
|
|
end
|
|
|
|
private
|
|
|
|
attr_reader :repository, :cache
|
|
|
|
delegate :raw_repository, to: :repository
|
|
|
|
def diverging_commit_counts(branch)
|
|
@root_ref_hash ||= raw_repository.commit(repository.root_ref).id
|
|
cache.fetch(:"diverging_commit_counts_#{branch.name}") do
|
|
number_commits_behind, number_commits_ahead =
|
|
raw_repository.diverging_commit_count(
|
|
@root_ref_hash,
|
|
branch.dereferenced_target.sha)
|
|
|
|
{ behind: number_commits_behind, ahead: number_commits_ahead }
|
|
end
|
|
end
|
|
end
|
|
end
|