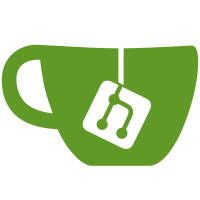
In order to implement https://gitlab.com/gitlab-org/gitlab-ee/issues/10179 we need several modifications on the CI config file. We are adding a new ports section in the default Image object. Each of these ports will accept: number, protocol and name. By default this new configuration will be only enabled in the Web IDE config file.
51 lines
1.2 KiB
Ruby
51 lines
1.2 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
module Gitlab
|
|
module Ci
|
|
module Build
|
|
class Image
|
|
attr_reader :alias, :command, :entrypoint, :name, :ports
|
|
|
|
class << self
|
|
def from_image(job)
|
|
image = Gitlab::Ci::Build::Image.new(job.options[:image])
|
|
return unless image.valid?
|
|
|
|
image
|
|
end
|
|
|
|
def from_services(job)
|
|
services = job.options[:services].to_a.map do |service|
|
|
Gitlab::Ci::Build::Image.new(service)
|
|
end
|
|
|
|
services.select(&:valid?).compact
|
|
end
|
|
end
|
|
|
|
def initialize(image)
|
|
if image.is_a?(String)
|
|
@name = image
|
|
@ports = []
|
|
elsif image.is_a?(Hash)
|
|
@alias = image[:alias]
|
|
@command = image[:command]
|
|
@entrypoint = image[:entrypoint]
|
|
@name = image[:name]
|
|
@ports = build_ports(image).select(&:valid?)
|
|
end
|
|
end
|
|
|
|
def valid?
|
|
@name.present?
|
|
end
|
|
|
|
private
|
|
|
|
def build_ports(image)
|
|
image[:ports].to_a.map { |port| ::Gitlab::Ci::Build::Port.new(port) }
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|