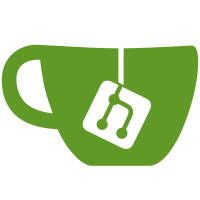
This refactors the AutocompleteController according to the guidelines and boundaries discussed in https://gitlab.com/gitlab-org/gitlab-ce/issues/49653. Specifically, ActiveRecord logic is moved to different finders, which are then used in the controller. View logic in turn is moved to presenters, instead of directly using ActiveRecord's "to_json" method. The finder MoveToProjectFinder is also adjusted according to the abstraction guidelines and boundaries, resulting in a much more simple finder. By using finders (and other abstractions) more actively, we can push a lot of logic out of the controller. We also remove the need for various "before_action" hooks, though this could be achieved without using finders as well. The various finders related to AutcompleteController have also been moved into a namespace. This removes the need for calling everything "AutocompleteSmurfFinder", instead you can use "Autocomplete::SmurfFinder".
37 lines
1.2 KiB
Ruby
37 lines
1.2 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
module Autocomplete
|
|
# Finder for retrieving a group to use for autocomplete data sources.
|
|
class GroupFinder
|
|
attr_reader :current_user, :project, :group_id
|
|
|
|
# current_user - The currently logged in user, if any.
|
|
# project - The Project (if any) to use for the autocomplete data sources.
|
|
# params - A Hash containing parameters to use for finding the project.
|
|
#
|
|
# The following parameters are supported:
|
|
#
|
|
# * group_id: The ID of the group to find.
|
|
def initialize(current_user = nil, project = nil, params = {})
|
|
@current_user = current_user
|
|
@project = project
|
|
@group_id = params[:group_id]
|
|
end
|
|
|
|
# Attempts to find a Group based on the current group ID.
|
|
def execute
|
|
return unless project.blank? && group_id.present?
|
|
|
|
group = Group.find(group_id)
|
|
|
|
# This removes the need for using `return render_404` and similar patterns
|
|
# in controllers that use this finder.
|
|
unless Ability.allowed?(current_user, :read_group, group)
|
|
raise ActiveRecord::RecordNotFound
|
|
.new("Could not find a Group with ID #{group_id}")
|
|
end
|
|
|
|
group
|
|
end
|
|
end
|
|
end
|