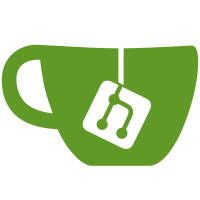
Previously `ProjectCacheWorker` would be scheduled once per ref, which would generate unnecessary I/O and load on Sidekiq, especially if many tags or branches were pushed at once. `ProjectCacheWorker` would expire three items: 1. Repository size: This only needs to be updated once per push. 2. Commit count: This only needs to be updated if the default branch is updated. 3. Project method caches: This only needs to be updated if the default branch changes, but only if certain files change (e.g. README, CHANGELOG, etc.). Because the third item requires looking at the actual changes in the commit deltas, we schedule one `ProjectCacheWorker` to handle the first two cases, and schedule a separate `ProjectCacheWorker` for the third case if it is needed. As a result, this brings down the number of `ProjectCacheWorker` jobs from N to 2. Closes https://gitlab.com/gitlab-org/gitlab-ce/issues/52046
138 lines
3.1 KiB
Ruby
138 lines
3.1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require 'spec_helper'
|
|
|
|
describe ::Gitlab::GitPostReceive do
|
|
set(:project) { create(:project, :repository) }
|
|
|
|
subject { described_class.new(project, "project-#{project.id}", changes.dup, {}) }
|
|
|
|
describe '#includes_branches?' do
|
|
context 'with no branches' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654321 210987 refs/nobranches/tag1
|
|
654322 210986 refs/tags/test1
|
|
654323 210985 refs/merge-requests/mr1
|
|
EOF
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(subject.includes_branches?).to be_falsey
|
|
end
|
|
end
|
|
|
|
context 'with branches' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654322 210986 refs/heads/test1
|
|
654321 210987 refs/tags/tag1
|
|
654323 210985 refs/merge-requests/mr1
|
|
EOF
|
|
end
|
|
|
|
it 'returns true' do
|
|
expect(subject.includes_branches?).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'with malformed changes' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
ref/heads/1 a
|
|
somebranch refs/heads/2
|
|
EOF
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(subject.includes_branches?).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
describe '#includes_tags?' do
|
|
context 'with no tags' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654321 210987 refs/notags/tag1
|
|
654322 210986 refs/heads/test1
|
|
654323 210985 refs/merge-requests/mr1
|
|
EOF
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(subject.includes_tags?).to be_falsey
|
|
end
|
|
end
|
|
|
|
context 'with tags' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654322 210986 refs/heads/test1
|
|
654321 210987 refs/tags/tag1
|
|
654323 210985 refs/merge-requests/mr1
|
|
EOF
|
|
end
|
|
|
|
it 'returns true' do
|
|
expect(subject.includes_tags?).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'with malformed changes' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
ref/tags/1 a
|
|
sometag refs/tags/2
|
|
EOF
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(subject.includes_tags?).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
describe '#includes_default_branch?' do
|
|
context 'with no default branch' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654321 210987 refs/heads/test1
|
|
654322 210986 refs/tags/#{project.default_branch}
|
|
654323 210985 refs/heads/test3
|
|
EOF
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(subject.includes_default_branch?).to be_falsey
|
|
end
|
|
end
|
|
|
|
context 'with a project with no default branch' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654321 210987 refs/heads/test1
|
|
EOF
|
|
end
|
|
|
|
it 'returns true' do
|
|
expect(project).to receive(:default_branch).and_return(nil)
|
|
expect(subject.includes_default_branch?).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'with default branch' do
|
|
let(:changes) do
|
|
<<~EOF
|
|
654322 210986 refs/heads/test1
|
|
654321 210987 refs/tags/test2
|
|
654323 210985 refs/heads/#{project.default_branch}
|
|
EOF
|
|
end
|
|
|
|
it 'returns true' do
|
|
expect(subject.includes_default_branch?).to be_truthy
|
|
end
|
|
end
|
|
end
|
|
end
|