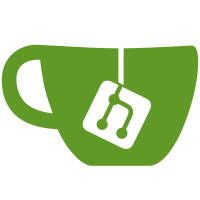
Given a subject Foo::Bar#baz mutant will run example groups with description starting with: - Foo::Bar#baz - Foo::Bar - Foo This basically means you can kill a mutation via a dedicated spec for a given method, all specs for a given object or all the specs within the object's namespace. In most of the cases you should target killing mutations via a dedicated spec for a particular method *however* there are valid cases where implicit coverage is absolutely fine.
85 lines
1.6 KiB
Ruby
85 lines
1.6 KiB
Ruby
# encoding: utf-8
|
|
|
|
module Mutant
|
|
class Killer
|
|
# Runner for rspec tests
|
|
class Rspec < self
|
|
|
|
private
|
|
|
|
# Run rspec test
|
|
#
|
|
# @return [true]
|
|
# when test is NOT successful
|
|
#
|
|
# @return [false]
|
|
# otherwise
|
|
#
|
|
# @api private
|
|
#
|
|
def run
|
|
mutation.insert
|
|
|
|
groups = example_groups
|
|
|
|
unless groups
|
|
$stderr.puts "No rspec example groups found for: #{match_prefixes.join(', ')}"
|
|
return false
|
|
end
|
|
|
|
reporter = RSpec::Core::Reporter.new
|
|
|
|
groups.each do |group|
|
|
return true unless group.run(reporter)
|
|
end
|
|
|
|
false
|
|
end
|
|
|
|
# Return match prefixes
|
|
#
|
|
# @return [Enumerble<String>]
|
|
#
|
|
# @api private
|
|
#
|
|
def match_prefixes
|
|
subject.match_prefixes
|
|
end
|
|
|
|
# Return example groups
|
|
#
|
|
# @return [Array<RSpec::Example>]
|
|
#
|
|
# @api private
|
|
#
|
|
def example_groups
|
|
match_prefixes.flat_map { |prefix| find_with(prefix) }.compact.uniq
|
|
end
|
|
|
|
# Return example groups that match expression
|
|
#
|
|
# @param [String] match_expression
|
|
#
|
|
# @return [Enumerable<String>]
|
|
#
|
|
# @api private
|
|
#
|
|
def find_with(match_expression)
|
|
all_example_groups.select do |example_group|
|
|
example_group.description.start_with?(match_expression)
|
|
end
|
|
end
|
|
|
|
# Return all example groups
|
|
#
|
|
# @return [Enumerable<RSpec::Example>]
|
|
#
|
|
# @api private
|
|
#
|
|
def all_example_groups
|
|
strategy.example_groups
|
|
end
|
|
|
|
end # Rspec
|
|
end # Killer
|
|
end # Mutant
|