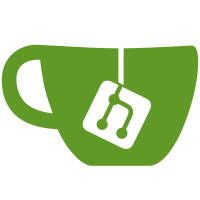
* Add Mutant::AST namespace to hold all AST related data / helpers. * Mutant::AST will be externalized into an ast-meta gem that can be shared with unparser for deduplication. * Over the time the mutators itself will not need to deal with semantic analysis of the AST anymore by themselves. * Move AST analysis for send nodes to AST::Meta * Fix #209
81 lines
1.6 KiB
Ruby
81 lines
1.6 KiB
Ruby
module Mutant
|
|
class Zombifier
|
|
# File containing source beeing zombified
|
|
class File
|
|
include Adamantium::Flat, Concord::Public.new(:path), AST::Sexp
|
|
|
|
# Zombify contents of file
|
|
#
|
|
# @return [self]
|
|
#
|
|
# @api private
|
|
#
|
|
def zombify(namespace)
|
|
$stderr.puts("Zombifying #{path}")
|
|
eval(
|
|
Unparser.unparse(namespaced_node(namespace)),
|
|
TOPLEVEL_BINDING,
|
|
path.to_s
|
|
)
|
|
self
|
|
end
|
|
|
|
# Find file by logical path
|
|
#
|
|
# @param [String] logical_name
|
|
#
|
|
# @return [File]
|
|
# if found
|
|
#
|
|
# @return [nil]
|
|
# otherwise
|
|
#
|
|
# @api private
|
|
#
|
|
def self.find(logical_name)
|
|
file_name =
|
|
case ::File.extname(logical_name)
|
|
when '.so'
|
|
return
|
|
when '.rb'
|
|
logical_name
|
|
else
|
|
"#{logical_name}.rb"
|
|
end
|
|
|
|
$LOAD_PATH.each do |path|
|
|
path = Pathname.new(path).join(file_name)
|
|
return new(path) if path.file?
|
|
end
|
|
|
|
$stderr.puts "Cannot find file #{file_name} in $LOAD_PATH"
|
|
nil
|
|
end
|
|
|
|
private
|
|
|
|
# Return node
|
|
#
|
|
# @return [Parser::AST::Node]
|
|
#
|
|
# @api private
|
|
#
|
|
def node
|
|
Parser::CurrentRuby.parse(path.read)
|
|
end
|
|
|
|
# Return namespaced root
|
|
#
|
|
# @param [Symbol] namespace
|
|
#
|
|
# @return [Parser::AST::Node]
|
|
#
|
|
# @api private
|
|
#
|
|
def namespaced_node(namespace)
|
|
s(:module, s(:const, nil, namespace), node)
|
|
end
|
|
|
|
end # File
|
|
end # Zombifier
|
|
end # Mutant
|