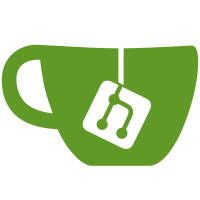
* Docs: named functions and function declarations * No more prototypal `extends`; update docs and example * More comprehensive documentation of the existential operator; closes #1631 * Better document operators, including `from` * No fat arrow class methods anymore * Destructuring shouldn’t say that default values are applied in case of undefined or null * Spinoff generator and async functions into their own sections; reorder things so that the sections on functions come just before classes, and destructuring goes next to the operators (which discuss assignment) * Rewrite “CoffeeScript 2” section, making it less practical and more explanatory; move practical info into “Usage” * Update “Variable Scoping and Lexical Safety” section to remove incorrect reference to Ruby (fixes #2360), add missing details about the safety wrapper, add note about `let`/`const`. * Updated browser compiler * Updated docs * Rewrite Literate CoffeeScript breaking changes * Split apart the “Breaking Changes” and “Unsupported Features” sections into separate sidebar items and files * Add example of `not in`, closes #3281 * Fix words in bold that should be in backticks * Consolidate some breaking changes sections * Add Node API documentation; closes #3551 * Move the chaining documentation out of the changelog into its own section
1.8 KiB
Operators and Aliases
Because the ==
operator frequently causes undesirable coercion, is intransitive, and has a different meaning than in other languages, CoffeeScript compiles ==
into ===
, and !=
into !==
. In addition, is
compiles into ===
, and isnt
into !==
.
You can use not
as an alias for !
.
For logic, and
compiles to &&
, and or
into ||
.
Instead of a newline or semicolon, then
can be used to separate conditions from expressions, in while
, if
/else
, and switch
/when
statements.
As in YAML, on
and yes
are the same as boolean true
, while off
and no
are boolean false
.
unless
can be used as the inverse of if
.
As a shortcut for this.property
, you can use @property
.
You can use in
to test for array presence, and of
to test for JavaScript object-key presence.
In a for
loop, from
compiles to the ES2015 of
. (Yes, it’s unfortunate; the CoffeeScript of
predates the ES2015 of
.)
To simplify math expressions, **
can be used for exponentiation and //
performs integer division. %
works just like in JavaScript, while %%
provides “dividend dependent modulo”:
codeFor('modulo')
All together now:
CoffeeScript | JavaScript |
---|---|
is |
=== |
isnt |
!== |
not |
! |
and |
&& |
or |
` |
true , yes , on |
true |
false , no , off |
false |
@ , this |
this |
a in b |
[].indexOf.call(b, a) >= 0 |
a of b |
a in b |
for a from b |
for (a of b) |
a ** b |
Math.pow(a, b) |
a // b |
Math.floor(a / b) |
a %% b |
(a % b + b) % b |
codeFor('aliases')