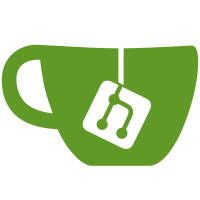
* Docs: named functions and function declarations * No more prototypal `extends`; update docs and example * More comprehensive documentation of the existential operator; closes #1631 * Better document operators, including `from` * No fat arrow class methods anymore * Destructuring shouldn’t say that default values are applied in case of undefined or null * Spinoff generator and async functions into their own sections; reorder things so that the sections on functions come just before classes, and destructuring goes next to the operators (which discuss assignment) * Rewrite “CoffeeScript 2” section, making it less practical and more explanatory; move practical info into “Usage” * Update “Variable Scoping and Lexical Safety” section to remove incorrect reference to Ruby (fixes #2360), add missing details about the safety wrapper, add note about `let`/`const`. * Updated browser compiler * Updated docs * Rewrite Literate CoffeeScript breaking changes * Split apart the “Breaking Changes” and “Unsupported Features” sections into separate sidebar items and files * Add example of `not in`, closes #3281 * Fix words in bold that should be in backticks * Consolidate some breaking changes sections * Add Node API documentation; closes #3551 * Move the chaining documentation out of the changelog into its own section
1.4 KiB
let
and const
: block-scoped and reassignment-protected variables
When CoffeeScript was designed, var
was intentionally omitted. This was to spare developers the mental housekeeping of needing to worry about variable declaration (var foo
) as opposed to variable assignment (foo = 1
). The CoffeeScript compiler automatically takes care of declaration for you, by generating var
statements at the top of every function scope. This makes it impossible to accidentally declare a global variable.
let
and const
add a useful ability to JavaScript in that you can use them to declare variables within a block scope, for example within an if
statement body or a for
loop body, whereas var
always declares variables in the scope of an entire function. When CoffeeScript 2 was designed, there was much discussion of whether this functionality was useful enough to outweigh the simplicity offered by never needing to consider variable declaration in CoffeeScript. In the end, it was decided that the simplicity was more valued. In CoffeeScript there remains only one type of variable.
Keep in mind that const
only protects you from reassigning a variable; it doesn’t prevent the variable’s value from changing, the way constants usually do in other languages:
const obj = {foo: 'bar'};
obj.foo = 'baz'; // Allowed!
obj = {}; // Throws error