mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
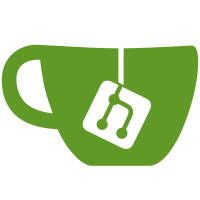
Implementation of https://github.com/docker/docker/issues/28319 Signed-off-by: Ben Firshman <ben@firshman.co.uk>
35 lines
879 B
Markdown
35 lines
879 B
Markdown
# Go client for the Docker Engine API
|
||
|
||
The `docker` command uses this package to communicate with the daemon. It can also be used by your own Go applications to do anything the command-line interface does – running containers, pulling images, managing swarms, etc.
|
||
|
||
For example, to list running containers (the equivalent of `docker ps`):
|
||
|
||
```go
|
||
package main
|
||
|
||
import (
|
||
"context"
|
||
"fmt"
|
||
|
||
"github.com/docker/docker/api/types"
|
||
"github.com/docker/docker/client"
|
||
)
|
||
|
||
func main() {
|
||
cli, err := client.NewEnvClient()
|
||
if err != nil {
|
||
panic(err)
|
||
}
|
||
|
||
containers, err := cli.ContainerList(context.Background(), types.ContainerListOptions{})
|
||
if err != nil {
|
||
panic(err)
|
||
}
|
||
|
||
for _, container := range containers {
|
||
fmt.Printf("%s %s\n", container.ID[:10], container.Image)
|
||
}
|
||
}
|
||
```
|
||
|
||
[Full documentation is available on GoDoc.](https://godoc.org/github.com/docker/docker/client)
|