mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
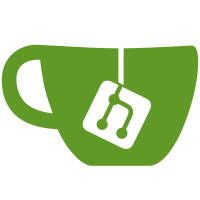
The "quiet" argument was only used in a single place (at daemon startup), and every other use had to pass "false" to prevent this function from logging warnings. Now that SysInfo contains the warnings that occurred when collecting the system information, we can make leave it up to the caller to use those warnings (and log them if wanted). Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
90 lines
2.5 KiB
Go
90 lines
2.5 KiB
Go
package runconfig // import "github.com/docker/docker/runconfig"
|
|
|
|
import (
|
|
"encoding/json"
|
|
"io"
|
|
|
|
"github.com/docker/docker/api/types/container"
|
|
networktypes "github.com/docker/docker/api/types/network"
|
|
"github.com/docker/docker/pkg/sysinfo"
|
|
)
|
|
|
|
// ContainerDecoder implements httputils.ContainerDecoder
|
|
// calling DecodeContainerConfig.
|
|
type ContainerDecoder struct {
|
|
GetSysInfo func() *sysinfo.SysInfo
|
|
}
|
|
|
|
// DecodeConfig makes ContainerDecoder to implement httputils.ContainerDecoder
|
|
func (r ContainerDecoder) DecodeConfig(src io.Reader) (*container.Config, *container.HostConfig, *networktypes.NetworkingConfig, error) {
|
|
var si *sysinfo.SysInfo
|
|
if r.GetSysInfo != nil {
|
|
si = r.GetSysInfo()
|
|
} else {
|
|
si = sysinfo.New()
|
|
}
|
|
|
|
return decodeContainerConfig(src, si)
|
|
}
|
|
|
|
// DecodeHostConfig makes ContainerDecoder to implement httputils.ContainerDecoder
|
|
func (r ContainerDecoder) DecodeHostConfig(src io.Reader) (*container.HostConfig, error) {
|
|
return decodeHostConfig(src)
|
|
}
|
|
|
|
// decodeContainerConfig decodes a json encoded config into a ContainerConfigWrapper
|
|
// struct and returns both a Config and a HostConfig struct
|
|
// Be aware this function is not checking whether the resulted structs are nil,
|
|
// it's your business to do so
|
|
func decodeContainerConfig(src io.Reader, si *sysinfo.SysInfo) (*container.Config, *container.HostConfig, *networktypes.NetworkingConfig, error) {
|
|
var w ContainerConfigWrapper
|
|
|
|
decoder := json.NewDecoder(src)
|
|
if err := decoder.Decode(&w); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
hc := w.getHostConfig()
|
|
|
|
// Perform platform-specific processing of Volumes and Binds.
|
|
if w.Config != nil && hc != nil {
|
|
|
|
// Initialize the volumes map if currently nil
|
|
if w.Config.Volumes == nil {
|
|
w.Config.Volumes = make(map[string]struct{})
|
|
}
|
|
}
|
|
|
|
// Certain parameters need daemon-side validation that cannot be done
|
|
// on the client, as only the daemon knows what is valid for the platform.
|
|
if err := validateNetMode(w.Config, hc); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
// Validate isolation
|
|
if err := validateIsolation(hc); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
// Validate QoS
|
|
if err := validateQoS(hc); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
// Validate Resources
|
|
if err := validateResources(hc, si); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
// Validate Privileged
|
|
if err := validatePrivileged(hc); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
// Validate ReadonlyRootfs
|
|
if err := validateReadonlyRootfs(hc); err != nil {
|
|
return nil, nil, nil, err
|
|
}
|
|
|
|
return w.Config, hc, w.NetworkingConfig, nil
|
|
}
|