mirror of
https://github.com/rails/rails.git
synced 2022-11-09 12:12:34 -05:00
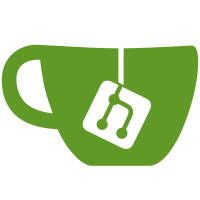
Added additional overview in n+1 queries that the scope is named with the rich text field name.
151 lines
5.2 KiB
Markdown
151 lines
5.2 KiB
Markdown
**DO NOT READ THIS FILE ON GITHUB, GUIDES ARE PUBLISHED ON https://guides.rubyonrails.org.**
|
||
|
||
Action Text Overview
|
||
====================
|
||
|
||
This guide provides you with all you need to get started in handling
|
||
rich text content.
|
||
|
||
After reading this guide, you will know:
|
||
|
||
* How to configure Action Text.
|
||
* How to handle rich text content.
|
||
* How to style rich text content.
|
||
|
||
--------------------------------------------------------------------------------
|
||
|
||
What is Action Text?
|
||
--------------------
|
||
|
||
Action Text brings rich text content and editing to Rails. It includes
|
||
the [Trix editor](https://trix-editor.org) that handles everything from formatting
|
||
to links to quotes to lists to embedded images and galleries.
|
||
The rich text content generated by the Trix editor is saved in its own
|
||
RichText model that's associated with any existing Active Record model in the application.
|
||
Any embedded images (or other attachments) are automatically stored using
|
||
Active Storage and associated with the included RichText model.
|
||
|
||
## Trix compared to other rich text editors
|
||
|
||
Most WYSIWYG editors are wrappers around HTML’s `contenteditable` and `execCommand` APIs,
|
||
designed by Microsoft to support live editing of web pages in Internet Explorer 5.5,
|
||
and [eventually reverse-engineered](https://blog.whatwg.org/the-road-to-html-5-contenteditable#history)
|
||
and copied by other browsers.
|
||
|
||
Because these APIs were never fully specified or documented,
|
||
and because WYSIWYG HTML editors are enormous in scope, each
|
||
browser's implementation has its own set of bugs and quirks,
|
||
and JavaScript developers are left to resolve the inconsistencies.
|
||
|
||
Trix sidesteps these inconsistencies by treating contenteditable
|
||
as an I/O device: when input makes its way to the editor, Trix converts that input
|
||
into an editing operation on its internal document model, then re-renders
|
||
that document back into the editor. This gives Trix complete control over what
|
||
happens after every keystroke, and avoids the need to use execCommand at all.
|
||
|
||
## Installation
|
||
|
||
Run `bin/rails action_text:install` to add the Yarn package and copy over the necessary migration. Also, you need to set up Active Storage for embedded images and other attachments. Please refer to the [Active Storage Overview](active_storage_overview.html) guide.
|
||
|
||
After the installation is complete, a Rails app using Webpacker should have the following changes:
|
||
|
||
1. Both `trix` and `@rails/actiontext` should be required in your JavaScript pack.
|
||
|
||
```js
|
||
// application.js
|
||
require("trix")
|
||
require("@rails/actiontext")
|
||
```
|
||
|
||
2. The `trix` stylesheet should be imported into `actiontext.scss`.
|
||
|
||
```scss
|
||
@import "trix/dist/trix";
|
||
```
|
||
|
||
Additionally, this `actiontext.scss` file should be imported into your stylesheet pack.
|
||
|
||
```scss
|
||
// application.scss
|
||
@import "./actiontext.scss";
|
||
```
|
||
|
||
## Examples
|
||
|
||
Adding a rich text field to an existing model:
|
||
|
||
```ruby
|
||
# app/models/message.rb
|
||
class Message < ApplicationRecord
|
||
has_rich_text :content
|
||
end
|
||
```
|
||
|
||
Note that you don't need to add a `content` field to your `messages` table.
|
||
|
||
Then refer to this field in the form for the model:
|
||
|
||
```erb
|
||
<%# app/views/messages/_form.html.erb %>
|
||
<%= form_with model: message do |form| %>
|
||
<div class="field">
|
||
<%= form.label :content %>
|
||
<%= form.rich_text_area :content %>
|
||
</div>
|
||
<% end %>
|
||
```
|
||
|
||
And finally, display the sanitized rich text on a page:
|
||
|
||
```erb
|
||
<%= @message.content %>
|
||
```
|
||
|
||
To accept the rich text content, all you have to do is permit the referenced attribute:
|
||
|
||
```ruby
|
||
class MessagesController < ApplicationController
|
||
def create
|
||
message = Message.create! params.require(:message).permit(:title, :content)
|
||
redirect_to message
|
||
end
|
||
end
|
||
```
|
||
|
||
## Avoid N+1 queries
|
||
|
||
If you wish to preload the dependent `ActionText::RichText` model, assuming your rich text field is named 'content', you can use the named scope:
|
||
|
||
```ruby
|
||
Message.all.with_rich_text_content # Preload the body without attachments.
|
||
Message.all.with_rich_text_content_and_embeds # Preload both body and attachments.
|
||
```
|
||
|
||
## Custom styling
|
||
|
||
By default, the Action Text editor and content is styled by the Trix defaults.
|
||
If you want to change these defaults, you'll want to remove
|
||
the `app/assets/stylesheets/actiontext.scss` linker and base your stylings on
|
||
the [contents of that file](https://raw.githubusercontent.com/basecamp/trix/master/dist/trix.css).
|
||
|
||
You can also style the HTML used for embedded images and other attachments (known as blobs).
|
||
On installation, Action Text will copy over a partial to
|
||
`app/views/active_storage/blobs/_blob.html.erb`, which you can specialize.
|
||
|
||
## API / Backend development
|
||
|
||
1. A backend API (for example, using JSON) needs a separate endpoint for uploading files that creates an `ActiveStorage::Blob` and returns its `attachable_sgid`:
|
||
|
||
```json
|
||
{
|
||
"attachable_sgid": "BAh7CEkiCG…"
|
||
}
|
||
```
|
||
|
||
2. Take that `attachable_sgid` and ask your frontend to insert it in rich text content using an `<action-text-attachment>` tag:
|
||
|
||
```html
|
||
<action-text-attachment sgid="BAh7CEkiCG…"></action-text-attachment>
|
||
```
|
||
|
||
This is based on Basecamp, so if you still can't find what you are looking for, check this [Basecamp Doc](https://github.com/basecamp/bc3-api/blob/master/sections/rich_text.md).
|