mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
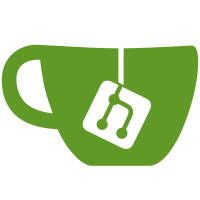
* lib/rake/cpu_counter.rb (count): prefer Etc.nprocessors, which is hundreds times faster. * test/rake/test_rake_cpu_counter.rb: add tests for features, and remove useless tests. git-svn-id: svn+ssh://ci.ruby-lang.org/ruby/trunk@48127 b2dd03c8-39d4-4d8f-98ff-823fe69b080e
68 lines
1.4 KiB
Ruby
68 lines
1.4 KiB
Ruby
require File.expand_path('../helper', __FILE__)
|
|
|
|
class TestRakeCpuCounter < Rake::TestCase
|
|
|
|
def setup
|
|
super
|
|
|
|
@cpu_counter = Rake::CpuCounter.new
|
|
end
|
|
|
|
def test_count
|
|
num = @cpu_counter.count
|
|
skip 'cannot count CPU' if num == nil
|
|
assert_kind_of Numeric, num
|
|
assert_operator num, :>=, 1
|
|
end
|
|
|
|
def test_count_with_default_nil
|
|
def @cpu_counter.count; nil; end
|
|
assert_equal(8, @cpu_counter.count_with_default(8))
|
|
assert_equal(4, @cpu_counter.count_with_default)
|
|
end
|
|
|
|
def test_count_with_default_raise
|
|
def @cpu_counter.count; raise; end
|
|
assert_equal(8, @cpu_counter.count_with_default(8))
|
|
assert_equal(4, @cpu_counter.count_with_default)
|
|
end
|
|
|
|
class TestClassMethod < Rake::TestCase
|
|
def setup
|
|
super
|
|
|
|
@klass = Class.new(Rake::CpuCounter)
|
|
end
|
|
|
|
def test_count
|
|
@klass.class_eval do
|
|
def count; 8; end
|
|
end
|
|
assert_equal(8, @klass.count)
|
|
end
|
|
|
|
def test_count_nil
|
|
counted = false
|
|
@klass.class_eval do
|
|
define_method(:count) do
|
|
counted = true
|
|
nil
|
|
end
|
|
end
|
|
assert_equal(4, @klass.count)
|
|
assert_equal(true, counted)
|
|
end
|
|
|
|
def test_count_raise
|
|
counted = false
|
|
@klass.class_eval do
|
|
define_method(:count) do
|
|
counted = true
|
|
raise
|
|
end
|
|
end
|
|
assert_equal(4, @klass.count)
|
|
assert_equal(true, counted)
|
|
end
|
|
end
|
|
end
|